Apex Test Class Examples for Web Services : jayakrishnasfdc
by: jayakrishnasfdc
blow post content copied from Jayakrishna Ganjikunta
click here to view original post
Before discussing about Test class for Web Services , if you want to get some background on Web Services
Please check out below Articles..
What is Web service and its different types
Apex REST Web services example

Generated code is saved as an Apex class containing the methods you can invoke for calling the web service. By default, test methods don’t support web service callouts, and tests that perform web service callouts fail.
To prevent tests from failing and to increase code coverage, Apex provides the built-in WebServiceMock interface and the Test.setMock method. Use WebServiceMock and Test.setMock to receive fake responses in a test method.
When you create an Apex class from a WSDL, the methods in the auto-generated class call WebServiceCallout.invoke, which performs the callout to the external service. When testing these methods, you can instruct the Apex runtime to generate a fake response whenever WebServiceCallout.invoke is called. To do so, implement the WebServiceMock interface and specify a fake response for the Apex runtime to send. Here are the steps in more detail.
Note:
- The class implementing the WebServiceMock interface can be either global or public.
- You can annotate this class with @isTest because it is used only in a test context. In this way, you can exclude it from your org’s code size limit of 6 MB.
Apex Test Classes will not let us conduct a HTTP callout; therefore, it cannot be used to test External APIs. However, there is a solution wherein Apex has an interface called HttpCalloutMock for standard callout tests.
Example: 1
Sample HTTPCallout Class:
public class AnimalLocator {
public static String getAnimalNameById(Integer id) {
Http http = new Http();
HttpRequest request = new HttpRequest();
request.setEndpoint(‘https://th-apex-http-callout.herokuapp.com/animals/’+id);
request.setMethod(‘GET’);
HttpResponse response = http.send(request);
String strResp = ”;
if (response.getStatusCode() == 200) {
Map < String, Object > results = (Map < String, Object >) JSON.deserializeUntyped(response.getBody());
Map < string, Object > animals = (Map < String, Object >) results.get(‘animal’);
strResp = string.valueof(animals.get(‘name’));
}
return strResp ;
}
}
Sample HTTP Mock Callout Class:
@isTest
global class AnimalLocatorMock implements HttpCalloutMock {
global HTTPResponse respond(HTTPRequest request) {
HttpResponse response = new HttpResponse();
response.setHeader(‘Content-Type’, ‘application/json’);
response.setBody(‘{“animal”: {“id”:2, “name”:”Test”}}’);
response.setStatusCode(200);
return response;
}
}
Test Class:
@isTest
private class AnimalLocatorTest {
static testMethod void testPostCallout() {
Test.setMock(HttpCalloutMock.class, new AnimalLocatorMock());
String strResp = AnimalLocator.getAnimalNameById(2);
}
}
Example: 2
global class DemoApexWebService { global class AccountInfo { WebService String AccName; WebService Integer AccNumber; } WebService static Account createAccount(AccountInfo info) { Account acct = new Account(); acct.Name = info.AccName; acct.AccountNumber = String.valueOf(info.AccNumber); insert acct; return acct; } } You can test the above with the following test class: @isTest private class TestNewWebService { static testMethod void testNewWebService() { DemoApexWebService.AccountInfo acc = new DemoApexWebService.AccountInfo(); acc.AccName = 'ABC'; acc.AccNumber = 123; DemoApexWebService.createAccount( acc ); Account[] a = [select AccountNumber from Account where AccountNumber = '123']; System.assertEquals(a.size(), 1); } }
Example : 3
Apex Class:
@RestResource(urlMapping=’/Cases/*’)
global with sharing class CaseManager {
@HttpGet
global static Case getCaseById() {
RestRequest request = RestContext.request;
// grab the caseId from the end of the URL
String caseId = request.requestURI.substring(
request.requestURI.lastIndexOf(‘/’)+1);
Case result = [SELECT CaseNumber,Subject,Status,Origin,Priority
FROM Case
WHERE Id = :caseId];
return result;
}
@HttpPost
global static ID createCase(String subject, String status,
String origin, String priority) {
Case thisCase = new Case(
Subject=subject,
Status=status,
Origin=origin,
Priority=priority);
insert thisCase;
return thisCase.Id;
}
@HttpDelete
global static void deleteCase() {
RestRequest request = RestContext.request;
String caseId = request.requestURI.substring(
request.requestURI.lastIndexOf(‘/’)+1);
Case thisCase = [SELECT Id FROM Case WHERE Id = :caseId];
delete thisCase;
}
@HttpPut
global static ID upsertCase(String subject, String status,
String origin, String priority, String id) {
Case thisCase = new Case(
Id=id,
Subject=subject,
Status=status,
Origin=origin,
Priority=priority);
// Match case by Id, if present.
// Otherwise, create new case.
upsert thisCase;
// Return the case ID.
return thisCase.Id;
}
@HttpPatch
global static ID updateCaseFields() {
RestRequest request = RestContext.request;
String caseId = request.requestURI.substring(
request.requestURI.lastIndexOf(‘/’)+1);
Case thisCase = [SELECT Id FROM Case WHERE Id = :caseId];
// Deserialize the JSON string into name-value pairs
Map params = (Map)JSON.deserializeUntyped(request.requestbody.tostring());
// Iterate through each parameter field and value
for(String fieldName : params.keySet()) {
// Set the field and value on the Case sObject
thisCase.put(fieldName, params.get(fieldName));
}
update thisCase;
return thisCase.Id;
}
}
Apex Test Class:
@IsTest
private class CaseManagerTest {
@isTest static void testGetCaseById() {
Id recordId = createTestRecord();
// Set up a test request
RestRequest request = new RestRequest();
request.requestUri =
‘https://yourInstance.salesforce.com/services/apexrest/Cases/’
+ recordId;
request.httpMethod = ‘GET’;
RestContext.request = request;
// Call the method to test
Case thisCase = CaseManager.getCaseById();
// Verify results
System.assert(thisCase != null);
System.assertEquals(‘Test record’, thisCase.Subject);
}
@isTest static void testCreateCase() {
// Call the method to test
ID thisCaseId = CaseManager.createCase(
‘Ferocious chipmunk’, ‘New’, ‘Phone’, ‘Low’);
// Verify results
System.assert(thisCaseId != null);
Case thisCase = [SELECT Id,Subject FROM Case WHERE Id=:thisCaseId];
System.assert(thisCase != null);
System.assertEquals(thisCase.Subject, ‘Ferocious chipmunk’);
}
@isTest static void testDeleteCase() {
Id recordId = createTestRecord();
// Set up a test request
RestRequest request = new RestRequest();
request.requestUri =
‘https://yourInstance.salesforce.com/services/apexrest/Cases/’
+ recordId;
request.httpMethod = ‘GET’;
RestContext.request = request;
// Call the method to test
CaseManager.deleteCase();
// Verify record is deleted
List cases = [SELECT Id FROM Case WHERE Id=:recordId];
System.assert(cases.size() == 0);
}
@isTest static void testUpsertCase() {
// 1. Insert new record
ID case1Id = CaseManager.upsertCase(
‘Ferocious chipmunk’, ‘New’, ‘Phone’, ‘Low’, null);
// Verify new record was created
System.assert(Case1Id != null);
Case case1 = [SELECT Id,Subject FROM Case WHERE Id=:case1Id];
System.assert(case1 != null);
System.assertEquals(case1.Subject, ‘Ferocious chipmunk’);
// 2. Update status of existing record to Working
ID case2Id = CaseManager.upsertCase(
‘Ferocious chipmunk’, ‘Working’, ‘Phone’, ‘Low’, case1Id);
// Verify record was updated
System.assertEquals(case1Id, case2Id);
Case case2 = [SELECT Id,Status FROM Case WHERE Id=:case2Id];
System.assert(case2 != null);
System.assertEquals(case2.Status, ‘Working’);
}
@isTest static void testUpdateCaseFields() {
Id recordId = createTestRecord();
RestRequest request = new RestRequest();
request.requestUri =
‘https://yourInstance.salesforce.com/services/apexrest/Cases/’
+ recordId;
request.httpMethod = ‘PATCH’;
request.addHeader(‘Content-Type’, ‘application/json’);
request.requestBody = Blob.valueOf(‘{“status”: “Working”}’);
RestContext.request = request;
// Update status of existing record to Working
ID thisCaseId = CaseManager.updateCaseFields();
// Verify record was updated
System.assert(thisCaseId != null);
Case thisCase = [SELECT Id,Status FROM Case WHERE Id=:thisCaseId];
System.assert(thisCase != null);
System.assertEquals(thisCase.Status, ‘Working’);
}
// Helper method
static Id createTestRecord() {
// Create test record
Case caseTest = new Case(
Subject=’Test record’,
Status=’New’,
Origin=’Phone’,
Priority=’Medium’);
insert caseTest;
return caseTest.Id;
}
}
January 02, 2021 at 11:49AM
Click here for more details...
=============================
The original post is available in Jayakrishna Ganjikunta by jayakrishnasfdc
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
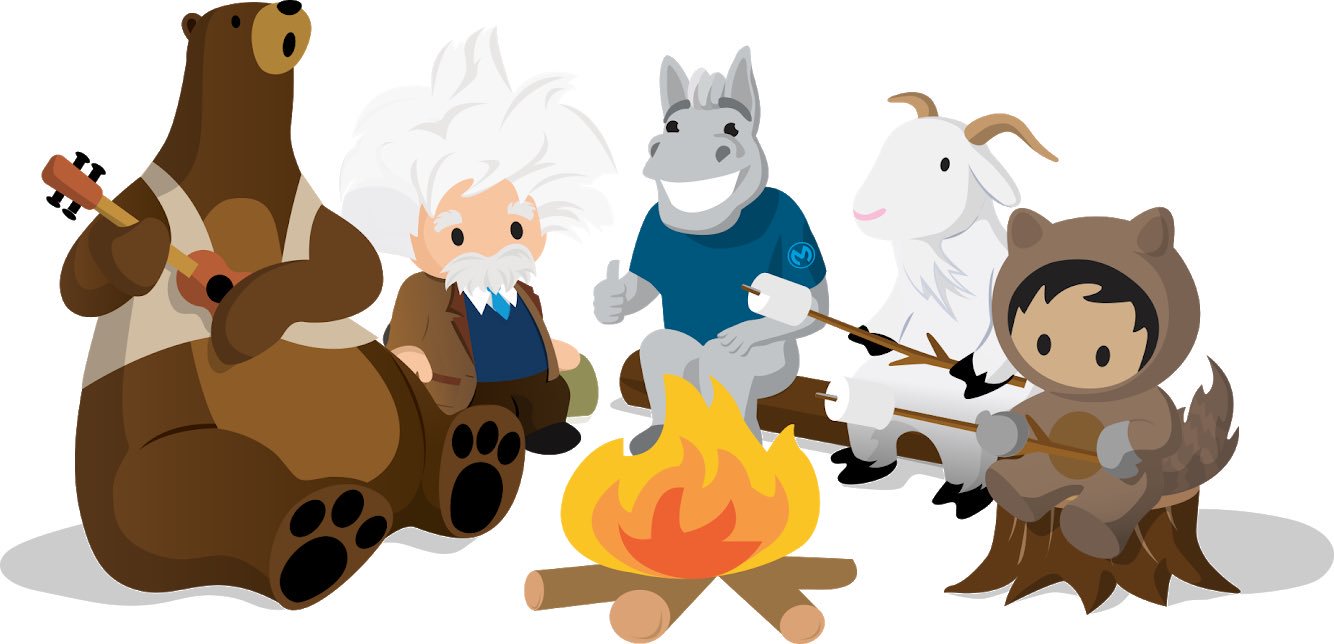
Post a Comment