Create a local user with password authentication for a SFTP-enabled storage account using an Azure PowerShell script : wmatthyssen
by: wmatthyssen
blow post content copied from Wim Matthyssen
click here to view original post
This blog post will demonstrate how to use an Azure PowerShell script to create a local user that uses password authentication for a SFTP-enabled storage account within a specific subscription.
These days, SFTP is also supported by blob storage, which means you can securely connect to Blob Storage using an SFTP endpoint. This allows you to utilize SFTP on a storage account for file access, transfer, and management purposes.
In a previous blog post, I already showed how you can create an Azure storage account with SFTP support enabled within a specific subscription, which you can find over here.
After you have enabled SFTP support for a specific storage account, you need to create a local user with the required access permissions to grant access to one or more root containers created on that storage account.
You need to create this local user because, up until now, Azure Storage doesn’t support shared access signature (SAS), or Azure Active Directory (Azure AD) authentication for accessing the SFTP endpoint.
At the moment, you can create and use a maximum of 1000 local users per storage account, and each created local user can access up to 100 containers.
You have the option to either use a password or a Secure Shell (SSH) public-private key pair as authentication methods for a local user you create. You can even configure both authentication forms for any or a specific local user you create and then let the connecting user choose which one to use. Just know that when you choose to allow the password method, you can’t set a custom password; Azure will generate one for you.
Next to that, you can only specify the read, write, list, delete, and create permissions on the container-level, because setting permissions at the directory level is not currently supported.
f you want to read some more about SFTP support for Azure Blob Storage, you can do so via the following Microsoft Docs link: SFTP support for Azure Blob Storage documentation
To automate the creation process of such a local user that uses the password authentication method, I wrote the below Azure PowerShell script, which does all of the following:
- Remove the breaking change warning messages.
- Create the C:\Temp folder if it does not already exist.
- Change the current context to the specified subscription.
- Create the local user with the required permissions and the password authentication method.
- Export the SSH password to txt file in C:\Temp folder.
To use the script, copy and save it as Create-Azure-Blob-Storage-SFTP-local-user-with-password-authentication.ps1 or download it from GitHub. Then, before using the script, adjust all variables to your needs, and then run the customized script from Windows Terminal, Visual Studio Code, or Windows PowerShell. Or you can simply run it from Cloud Shell.
Prerequisites
- An Azure subscription (preferably more than one if you want to follow the Enterprise-Scale architecture).
- An Azure Administrator account with the necessary RBAC roles.
- An existing storage account with SFTP support enabled.
- Version 9.4.0 of the Azure Az PowerShell module is required.
- Change all the variables in the script where needed to fit your needs (you can find an adjusted example in one of the screenshots below).


Azure PowerShell script
If you are not running the script from Cloud Shell, don’t forget to sign in with the Connect-AzAccount cmdlet to connect your Azure account.

You can then run the script with the required parameters:
.\Create-Azure-Blob-Storage-SFTP-local-user-with-password-authentication -SubscriptionName <"your Azure subscription name here"> -UserName <"your local user name here"> -ContainerName <"your storage acocunt container name here">

<#
.SYNOPSIS
A script used to create a specified local user that uses password authentication for use with a storage account with the SSH File Transfer Protocol (SFTP) enabled.
.DESCRIPTION
A script used to create a specified local user that uses password authentication for use with a storage account with the SSH File Transfer Protocol (SFTP) enabled.
This script will do all of the following:
Remove the breaking change warning messages.
Create the C:\Temp folder if it does not already exist.
Change the current context to the specified subscription.
Create the local user with the required permissions and the password authentication method.
Export the SSH password to a txt file in the C:\Temp folder.
.NOTES
Filename: Create-Azure-Blob-Storage-SFTP-local-user-with-password-authentication.ps1
Created: 04/04/2023
Last modified: 18/04/2023
Author: Wim Matthyssen
Version: 1.1
PowerShell: Azure PowerShell and Azure Cloud Shell
Requires: PowerShell Az (v9.4.0)
Action: Change variables were needed to fit your needs.
Disclaimer: This script is provided "as is" with no warranties.
.EXAMPLE
Connect-AzAccount
Get-AzTenant (if not using the default tenant)
Set-AzContext -tenantID "xxxxxxxx-xxxx-xxxx-xxxxxxxxxxxx" (if not using the default tenant)
.\Create-Azure-Blob-Storage-SFTP-local-user-with-password-authentication -SubscriptionName <"your Azure subscription name here"> -UserName <"your local user name here"> -ContainerName <"your storage acocunt container name here">
-> .\Create-Azure-Blob-Storage-SFTP-local-user-with-password-authentication.ps1 -SubscriptionName sub-prd-myh-corp-01 -ContainerName file-upload -userName wmsftp01
.LINK
https://wmatthyssen.com/2023/04/18/create-a-local-user-with-password-authentication-for-a-sftp-enabled-storage-account-using-an-azure-powershell-script/
#>
## ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
## Parameters
param(
# $subscriptionName -> Name of the Azure Subscription
[parameter(Mandatory =$true)][ValidateNotNullOrEmpty()] [string] $subscriptionName,
# $containerName -> Name of the container
[parameter(Mandatory =$true)][ValidateNotNullOrEmpty()] [string] $containerName,
# $userName -> Name of the user
[parameter(Mandatory =$true)][ValidateNotNullOrEmpty()] [string] $userName
)
## ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
## Variables
$tempFolderName = "Temp"
$tempFolder = "C:\" + $tempFolderName +"\"
$itemType = "Directory"
$rgNameStorage = #<your storage account resource group name here> The name of the Azure resource group in which your new or existing storage account is deployed. Example: "rg-hub-myh-storage-01"
$storageAccountName = #<your storage account name here> The name of your new storage account. Example: "stprdmyhsftp01"
$global:currenttime= Set-PSBreakpoint -Variable currenttime -Mode Read -Action {$global:currenttime= Get-Date -UFormat "%A %m/%d/%Y %R"}
$foregroundColor1 = "Green"
$foregroundColor2 = "Yellow"
$writeEmptyLine = "`n"
$writeSeperatorSpaces = " - "
## ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
## Remove the breaking change warning messages
Set-Item -Path Env:\SuppressAzurePowerShellBreakingChangeWarnings -Value $true | Out-Null
Update-AzConfig -DisplayBreakingChangeWarning $false | Out-Null
## ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
## Write script started
Write-Host ($writeEmptyLine + "# Script started. Without errors, it can take up to 4 minutes to complete" + $writeSeperatorSpaces + $currentTime)`
-foregroundcolor $foregroundColor1 $writeEmptyLine
## ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
## Create the C:\Temp folder if it does not already exist
If(!(test-path $tempFolder))
{
New-Item -Path "C:\" -Name $tempFolderName -ItemType $itemType -Force | Out-Null
}
Write-Host ($writeEmptyLine + "# $tempFolderName folder available" + $writeSeperatorSpaces + $currentTime)`
-foregroundcolor $foregroundColor2 $writeEmptyLine
## ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
## Change the current context to the specified subscription
$subName = Get-AzSubscription | Where-Object {$_.Name -like $subscriptionName}
Set-AzContext -SubscriptionId $subName.SubscriptionId | Out-Null
Write-Host ($writeEmptyLine + "# Specified subscription in current tenant selected" + $writeSeperatorSpaces + $currentTime)`
-foregroundcolor $foregroundColor2 $writeEmptyLine
## ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
## Create the local user with the required permissions and the password authentication method
$permissionScope1 = New-AzStorageLocalUserPermissionScope -Permission wl -Service blob -ResourceName $containerName
Set-AzStorageLocalUser -ResourceGroupName $rgNameStorage -AccountName $storageAccountName -UserName $userName -HasSshPassword $true `
-HomeDirectory $containerName -PermissionScope $permissionScope1 | Out-Null
# Regenerate the SSH password of the specified local user
$sshPassword = New-AzStorageLocalUserSshPassword -ResourceGroupName $rgNameStorage -AccountName $storageAccountName -UserName $userName
Write-Host ($writeEmptyLine + "# Local user $userName created with SSH Password and required container permissions" + $writeSeperatorSpaces + $currentTime)`
-foregroundcolor $foregroundColor2 $writeEmptyLine
## ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
## Export the SSH password to a txt file in the C:\Temp folder
$sshPassword | Out-File C:\Temp\$userName.txt
Write-Host ($writeEmptyLine + "# SSH Password exported to $userName.txt in the C:\Temp folder" + $writeSeperatorSpaces + $currentTime)`
-foregroundcolor $foregroundColor2 $writeEmptyLine
## ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
## Write script completed
Write-Host ($writeEmptyLine + "# Script completed" + $writeSeperatorSpaces + $currentTime)`
-foregroundcolor $foregroundColor1 $writeEmptyLine
## ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------






I hope this Azure PowerShell script is useful for you and can help you create a local user for any SFTP-enabled storage account in your Azure environment.
If you have any questions or recommendations about it, feel free to contact me through my Twitter handle (@wmatthyssen) or to just leave a comment.
April 18, 2023 at 04:19PM
Click here for more details...
=============================
The original post is available in Wim Matthyssen by wmatthyssen
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
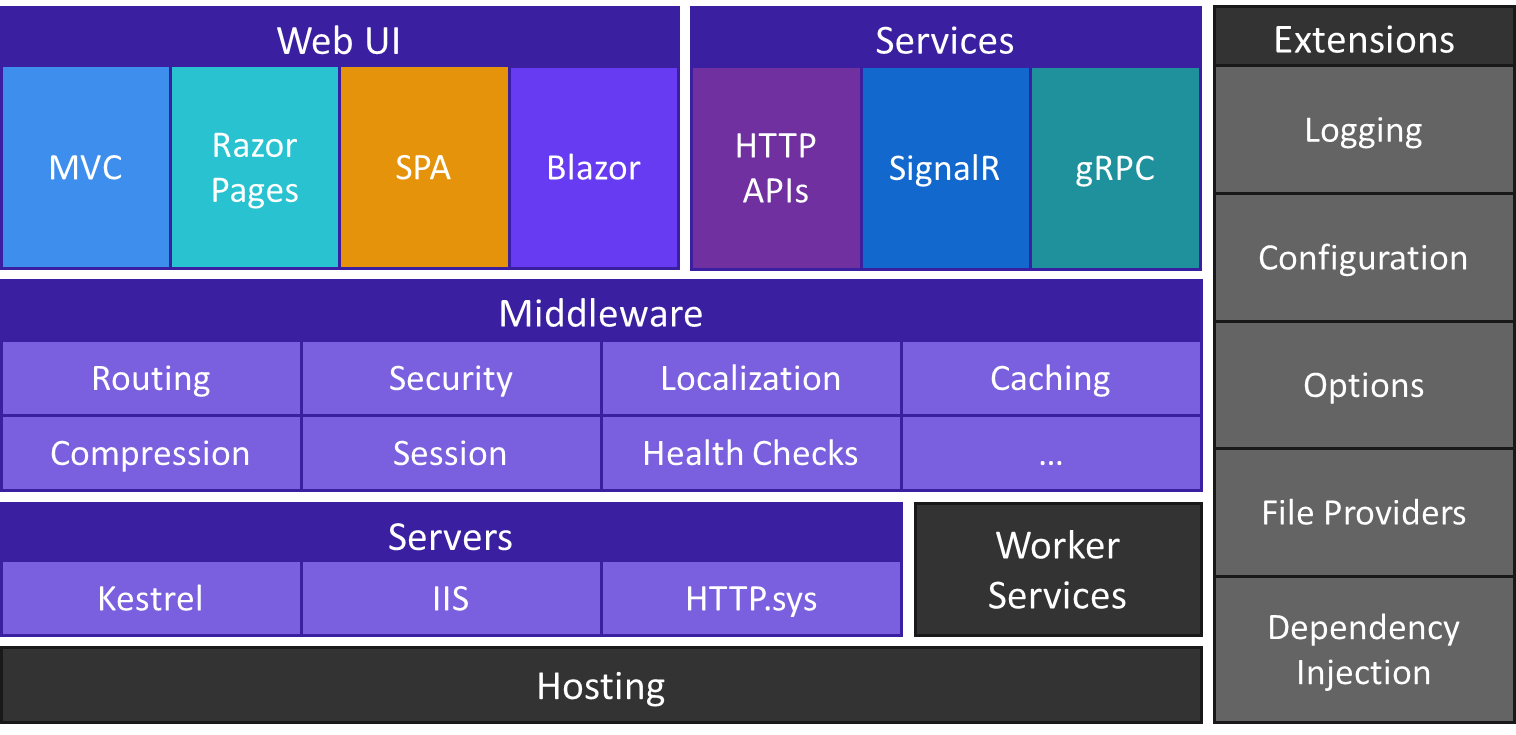
Post a Comment