Audio Notes: Creating an Interface to Browse Content : jamie_maguire
by: jamie_maguire
blow post content copied from Jamie Maguire
click here to view original post
In an earlier blog post, I introduced Audio Notes.
This is a new a SaaS experiment that uses artificial intelligence, speech to text, and text analytics to automatically summarise audio and create concise notes from your recordings.
You can find more information about the project in these earlier blog posts. These set the scene and show which Azure AI services make this end-to-end solution possible:
- Introduction
- Using Azure AI Speech to Perform Continual Speech to Text
- Transcription Using Azure AI Language to Perform Document Summarization
- Blending Azure AI Speech and Azure Language to Create a Micro SaaS
In this blog post (Part 5), a UI is created to view existing audio notes and to create a new audio note.
Read on to see how all this hangs together.
~
Desired Output
A simple grid layout with tiles is needed. Each tile will display the title of the audio note and a short sentence about the content.
Something like this will do:
Shout out to Ron Fields for creating this CodePen.
~
Layout Page
The existing layout/template looks like it was created by a backend developer!
So, it’s over to the bootstrap site to get a slightly better layout with a sidebar:
After updating the main layout.cshtml page in the Audio Notes solution, we a slightly better interface:
The tile layout of audio notes will be displayed when you click on the link Your Notes. First, we need to adjust the existing code to create batches of audio notes.
~
Adjusting Code to Return Batches of Audio Notes
We need to adjust the code to return batches of collections. Each batch will contain 3 audio notes per row.
An extension method is created to create batches of collections from a list:
public static IEnumerable<IEnumerable<T>> Batch<T>(this IEnumerable<T> enumerator, int size) { var length = enumerator.Count(); var pos = 0; do { yield return enumerator.Skip(pos).Take(size); pos = pos + size; } while (pos < length); }
Kudos to Brian Penderson for writing this helpful method.
~
Speech Notes View Model
View models of type SpeechNotesViewModel are bound to the UI. You can see a definition of this view model here:
public class SpeechNotesViewModel { public List<SpeechNoteViewModel> SpeechNotes { get; set; } public IEnumerable<IEnumerable<SpeechNoteViewModel>> SpeechNoteBatches { get; set; } public SpeechNotesViewModel() { this.SpeechNotes = new List<SpeechNoteViewModel>(); this.SpeechNoteBatches = new List<IEnumerable<SpeechNoteViewModel>>(); } }
The property SpeechNotes contains the raw list of speech notes.
The property SpeechNotesBatches contains batches of speech notes.
GetSpeechNotesData
A helper method is created for to support quick testing:
public void GetSpeechNotesData() { SpeechNotesViewModel speechNotesData = new SpeechNotesViewModel(); speechNotesData.SpeechNotes.Add(new SpeechNoteViewModel { Color = SpeechNoteViewModel.ColorType.Blue, Title = "Note 1 Title", Description = "Note 1" }); speechNotesData.SpeechNotes.Add(new SpeechNoteViewModel { Color = SpeechNoteViewModel.ColorType.Orange, Title = "Note 2 Title", Description = "Note 2" }); speechNotesData.SpeechNotes.Add(new SpeechNoteViewModel { Color = SpeechNoteViewModel.ColorType.Green, Title = "Note 3 Title", Description = "Note 3" }); speechNotesData.SpeechNotes.Add(new SpeechNoteViewModel { Color = SpeechNoteViewModel.ColorType.Yellow, Title = "Note 4 Title", Description = "Note 4" }); speechNotesData.SpeechNotes.Add(new SpeechNoteViewModel { Color = SpeechNoteViewModel.ColorType.Red, Title = "Note 5 Title", Description = "Note 5" }); speechNotesData.SpeechNotes.Add(new SpeechNoteViewModel { Color = SpeechNoteViewModel.ColorType.Purple, Title = "Note 6 Title", Description = "Note 6" }); this.SpeechNotes = speechNotesData.SpeechNotes; this.SpeechNoteBatches = speechNotesData.SpeechNotes.Batch(3); }
Six notes are added. The properties Color, Title, and Description are also set.
These are used to create batches.
As there are only 6 audio notes in the master collection, there will be only 2 batches in the property SpeechNoteBatches.
HTML to Render Audio Notes– YourNotes.cshtml
The following HTML is used to render all audio notes:
@model AudioNotes.Web.ViewModels.SpeechNotesViewModel @{ } @{ Layout = "~/Pages/Shared/_Layout.cshtml"; } <div class="container"> <div class="col-md-12"> @foreach (var batch in Model.SpeechNoteBatches) { @Html.Partial("_NoteTile", batch) } </div> </div>
You’ll see from the above, we loop through each batch and render a partial view _NoteTile. Here is the definition of this partial view:
@model IEnumerable<AudioNotes.Web.ViewModels.SpeechNoteViewModel> @foreach (var note in Model) { <a href="http://www.ubh.com" class="tile @note.Color"> <h3 class="title">@note.Title</h3> <p>@note.Description</p> </a> }
Nothing too fancy in the above HTML. We set the colour, title, and description. With everything in place, we can test the new updates.
~
Testing the Updates
Launching the application renders the new bootstrap layout with the sidebar:
We can click on the Your Notes link and we’re taken to the new tile layout. All 6 audio notes are rendered across 2 batches:
Looking better. The colour property isn’t being picked up, however. We can tackle that a later time.
~
Next Steps
The capture note screen will need modified to use the new look and feel. The input and output fields will also need revised. It looks bad!
ASK – If you have ideas or fancy redoing this HTML then I will happily incorporate these into the HTML.
~
Summary
In this blog, we’ve improved the look and feel of the Audio Notes SaaS experiment.
In the next part of this miniseries, we’ll update the note capture screen.
Further Reading and Resources
The following resources will give you more information as to how this solution was developed and show you the previous SaaS Daily Tracker was created.
- Audio Notes Part 1 – Introduction
- Audio Notes Part 2 – Using Azure AI Speech to Perform Continual Speech to Text Transcription
- Audio Notes Part 3 – Using Azure AI Language to Perform Document Summarization
- Audio Notes Part – Blending Azure AI Speech and Azure Language to Create a Micro SaaS
- How To: Creating a Private Journal and Mood Tracking App Using .NET 6, Chat GPT, C# and Visual Studio 2022
- From Prototype to Production: Turning the Daily Tracker Journal and Mood Tracker App into a Micro SaaS
- Announcement: Journal and Mood Tracking Micro-SaaS Released
- Azure AI Speech Services
- Azure AI Language Services
October 21, 2023 at 12:30PM
Click here for more details...
=============================
The original post is available in Jamie Maguire by jamie_maguire
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
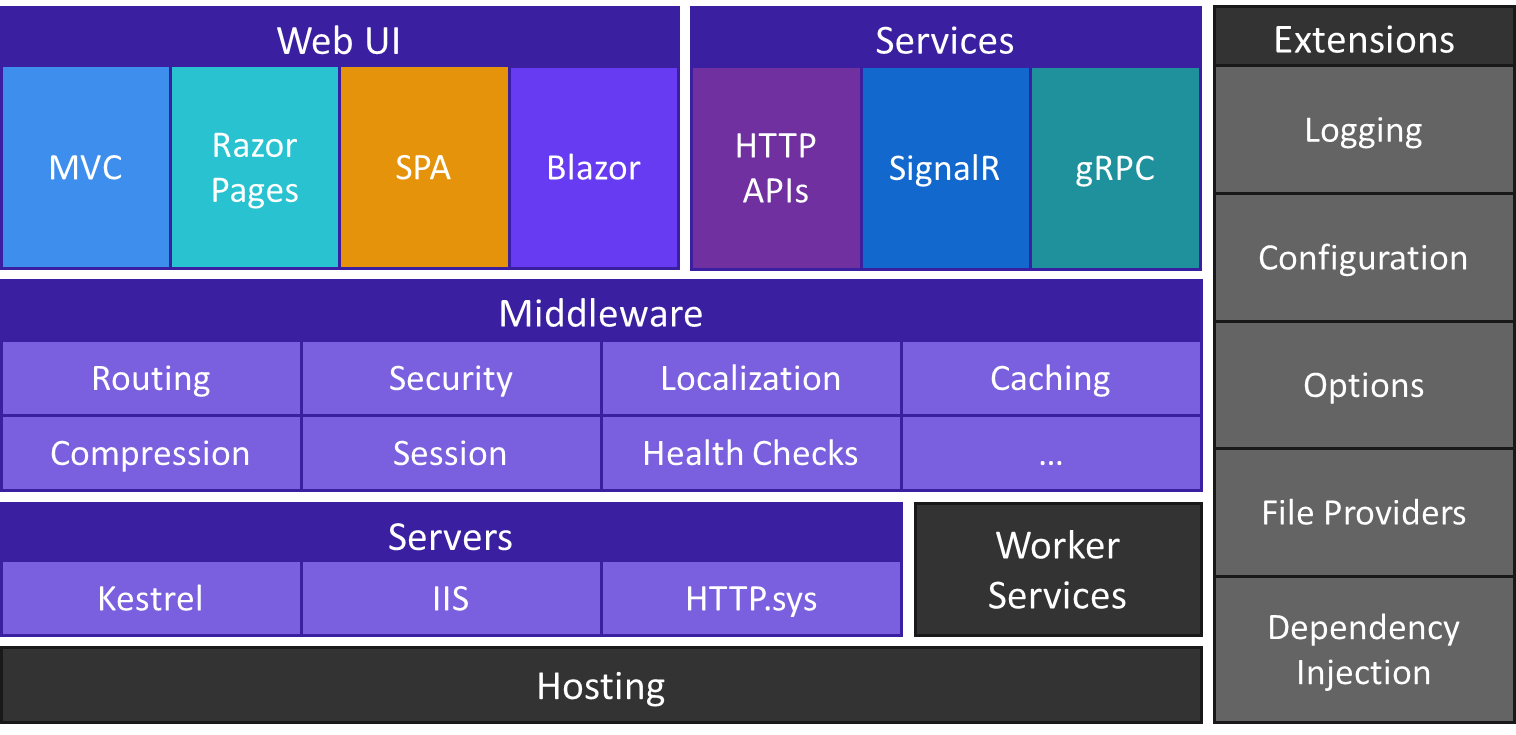
Post a Comment